Web apps that last
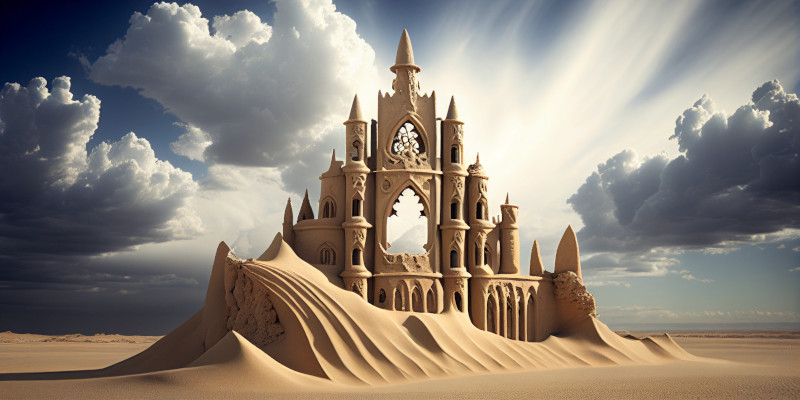
When you're building a new web application, or even a new feature, how can you ensure that you're not creating a nightmare code base that will need to be rewritten completely in a few years?
Some people will say it's hopeless to even try and write code that will last. I've even heard people suggest that you should aim to rewrite all your code every few years. That sounds like a very expensive, wasteful strategy.
In two decades of building web apps, I've seen many codebases start as a shiny new prototype and grow into a huge system. In some cases, they've become old, ugly, painful legacy systems that teams are begging to rewrite and replace. (And often those rewrites will themselves grow into ugly, painful legacy systems!) But sometimes a codebase will remain more or less unchanged a decade later, running smoothly as ever.
I believe there are some decisions you can make when writing code that will help it to last longer, and withstand the test of time.
Change is inevitable
Probably the one thing you can be sure of is that change will come. The goals of a business will change, and the people within a business will change. There will inevitably be features added, and existing features will evolve and be repurposed. The names of the products will almost surely change. So is it even possible to write code that doesn't need to change?
I think the key is in the phrase "If it ain't broke, don't fix it". Code that is fulfilling its task, that is doing what it's supposed to do, and is bug-free, is code that will last a long time.
Nightmare code
To understand how to write code that will last, let's think about the opposite: a nightmare codebase that demands to be rewritten. The worst I've seen is a web server written as a giant single file with thousands of lines of code. A system built like a house of cards, where changing one thing will break everything. Code that is very difficult to read or understand. Code that literally gives developers nightmares.
Unfortunately, this is often the kind of code that comes out of throwing together a quick prototype. A hero developer stays up late one night and churns out a first draft like a stream-of-consciousness. The next morning, the business owner is delighted to see their dreams come to life. Everyone's happy.
Then, they ask to change just one thing. Add this little feature. And this other feature. And now this user needs this other thing. And could you just change that other thing quick?
Months later, and this rough draft has accidentally become the foundation for a web application that continues to grow, held together with digital duct tape.
So how do you prevent this nightmare from unfolding?
Do one thing, and do it well
Modularity is extremely important in writing code that will last. And a good module is a piece of code that does one thing, and does it well. That one thing might be interfacing with a single database table. Or it could be handling HTTP calls on a single URL and passing the data to and from other modules that talk to the database.
I find generally that it works best when each module has zero, one or two major dependencies. With zero dependencies, you have a set of functions that receive input data, process it in some way, and return results. With one dependency, you have a set of functions that act as an abstraction or interface to that dependency. With two dependencies, you're writing code that bridges the gap between the two, acting as an adapter or controller.
More than two major dependencies, and you should ask yourself if there's any way to split things up into smaller pieces that are responsible for fewer things.
A dependency might not always be a program you have to install. Another module in your system is also a dependency. So is your business logic. I think about dependencies as anything that your module "knows about". This could even be the shape of certain data structures that might not have explicit type definitions.
The fewer things a module knows about, the more likely the module will be able to persist unchanged over time, because there will be fewer reasons to change it.
When your web application is built with small, independent modules that only do one thing, the chances are much, much lower that any of those pieces will need to be rewritten. And the chance of the whole application needing to be rewritten all at once drops to nearly zero. Even if you later want to do a major redesign, you'll find it easier to copy over lots of these older, simple modules to reuse in the new system.
Finally, a tangible example
Let's say you need to send out a Forgot Password email. You could do the whole thing in one file, but I would prefer to split it up like this:
A module that knows how to actually send an email using AWS SES or something, but doesn't know the recipient, subject or body of the email.
function sendEmail(toAddress, subject, body)
, for example.A module that knows about the subject and body of the Forgot Password email, but doesn't know who it's sending to or what the reset URL will be.
function sendForgotPasswordEmail(toAddress, resetUrl)
A module for the user table in the database, that has a function to generate a reset code, but doesn't know how the reset code will be used or even whether an email will be sent out.
function createResetCode(userEmail)
A module that knows about the URL structure of the site, and has a function that can generate a password reset link from a reset code.
function getResetUrlFromCode(code)
A module that ties everything together. It takes an email address, calls
createResetCode
, uses that to callgetResetUrlFromCode
, passes that togetForgotPasswordEmail
, and sends the recipient address and email body tosendForgotPasswordEmail
.forgotPassword(email)
A user interface widget with a form, a text field and a button, so the user can type in their email address and click
Send password reset link
. When the form is submitted, it tells the user to go wait for the email.A module that is responsible for the server-side password reset part of the system. It receives the form submission, pulls the email address from the form data, calls
forgotPassword
, and then sends asuccess
status back to the browser.
Here, only a few modules are likely to change. You'll probably see changes to the sendForgotPasswordEmail
function, as well as the user interface widget. All the other modules I've outlined are very reusable, and highly unlikely to change, unless you change your email sending provider, or your database software, or something else major. Even in those situations, the code that needs to change is very isolated and easy to replace without affecting anything else.
You can even improve on this further, by having the contents of the email be database-driven, so that non-technical staff members can change the email templates themselves through an admin interface. But an architecture like this is a good starting point that makes those sorts of changes simpler to make.
A good start
If you get in the habit of writing more modular code, and splitting things up as early as possible, then the next time you're throwing together a quick prototype, you'll be able to lean on those principals in the process.
Instead of a giant ball of tangled dependencies and logic, you'll be building smaller, simpler, reusable components that can be used as solid building blocks. Some of these will be so useful and generic that you'll even be able to reuse them in completely different systems without changing them at all.