Journaling for thirty years
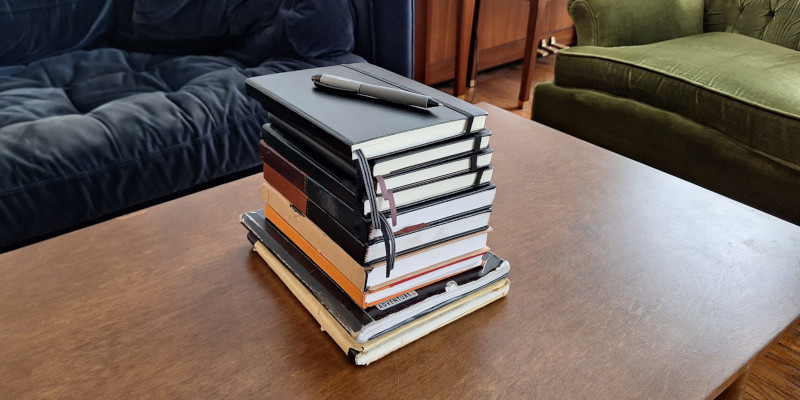
I've been writing in my journal since I was sixteen. I just turned forty-four, so that means I have journals covering almost thirty years of my life.
When other people hear about my journaling practice, they say things like "I could never do that", or "I tried it but I couldn't keep up with it."
So if you, too, would like to keep a journal but are struggling to start or maintain it, I thought I'd talk about how I've managed to do it consistently for so long.
First of all, for me, it is definitely not a daily practice! I absolutely do not care how often I do it. Sometimes I write more than once in a day. Sometimes I write a couple times in a month. It doesn't matter to me. This is because..
I don't try to write about everything that happened. Yes, I do write about some things that happened, but these may or may not be big things. I write about the big things, sure, but I also write a lot about small, insignificant anecdotes that I'd like to remember. But most of what I write isn't even about things that happened. Instead...
I write about whatever is on my mind. I use journaling to dump my thoughts on to paper, to give myself a time and place to process the things going on in my life, and help me to put into words the feelings and worries and hopes and goings-ons in my world at that moment. Whether I'm puzzling over which direction to go in my business, or processing a big social event I just attended, or thinking about some world event, or documenting the unfolding pandemic, or taking stock of those special moments as a parent, my journal is a place where I can "think out loud" (on paper).
I write as little or much as I feel like. Sometimes I write just a few sentences. Sometimes I write five pages. If I feel like stopping, I stop. If I get pulled away to do something else, I may or may not come back to finish later. It doesn't really matter. I don't try to write a full page, even though the journals I use have a place for the date at the top. I'll stop and start on any line.
When I start writing, I write the date. When I'm done, I sign my name and close the book. It's not important, but I did this as a teenager for whatever reason, and this gives a sense of closure, like the thoughts are now frozen in the book and I can walk away and leave them behind.
I can go back and see how I felt at certain times in my past. For example, my wife is pregnant with our second son, so I was recently going back to reading what was going on in my mind when she was pregnant with our first son. It was surprising to be reminded of all the stuff that was going on back then that I'd totally forgotten about. It's always enlightening to read about the things I was worried about that never came to be. And it's fun to read about decisions I made while journaling that ended up having a significant impact on my life for years to come. It's also interesting to see the patterns and cycles, the ups and downs in my life, my business, and in my energy levels and inspiration, that ebb and flow year after year.
Even though its nice to go back and read old journals, almost all the benefit comes from the journaling itself. It's the process of thinking, reflecting and writing that takes the thoughts out of my head and puts them on paper. When I'm done journaling, it's like a fresh start. I'm decluttering my mind, getting my thoughts in order, and throwing out things I no longer need. When I'm done journaling, I have a fresh energy where I'm ready to take on something new.
Although I don't have any strict routine around journaling, I almost always like to journal on Saturday mornings with my first coffee of the day. It's a great time to have some quiet time to myself, wake up slowly, reflect on what happened over the past week, take stock of all that's going on in the back of my mind, and decide on what I want to do with my free time on the weekend. I'll sit there for an hour or so, and when I finish, I'm relaxed and inspired and ready to go have a wonderful weekend.
I don't write for anyone but myself. Sure, I know in the back of my mind that one day, in the far future, my children or grandchildren might read through these, so there are a few things I might not say, or might write in a secret code for myself, but otherwise these are secret documents that are written for nobody but myself.
It doesn't really matter what books or pens you use, as long as you enjoy them. My favourite books are either Moleskine or Leuchturm1917, A5 ruled black hardcover notebooks. They're pricey, but they don't fall apart, and they're a pleasure to write in. I want them to last a long time. My favourite pen at the moment is a retractable black Stabilo Palette 0.4mm gel pen.
If you're curious about journaling, or struggled with it in the past, I hope this helps you see a different approach that might work better for you. I highly recommend journaling, though it might not be for everyone. For me, it helps me think and process my life, allowing my mind to be quieter overall, while having the small benefit of documenting the things in my life that would otherwise be easily forgotten.